Validate inputs & outputs
You can use validator functions to validate inputs, similar to the platform validators. Validation functions have full access to the UI and to the context of the script. For example, you can create a function to validate min-max values, assuring that the minimum value is less than the maximum value.
In addition, RichFunctionView supports output values validation.
Validation functions should be written in JavaScript to avoid client-server communication delays.
Validation functions have a single input and a single output.
params
object may have arbitrary data for the validator to behave differently in certain situations.validatorFunc
object is a JS function (e.g. arrow function) that actually will be called each time the input is changed.
Here is an example used in the default Object cooling script.
- Result
- Validator function
- Assigning validator to a param
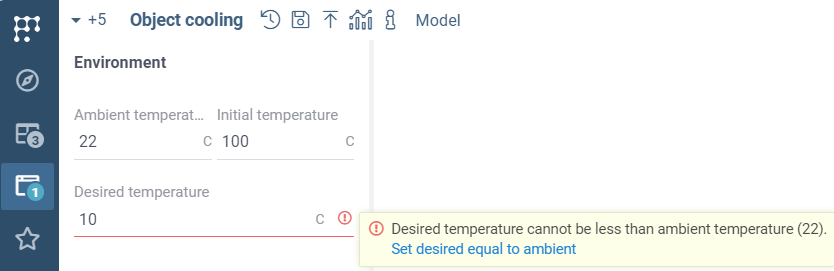
//name: DesiredTempValidator
//input: object params
//output: object validator
export function DesiredTempValidator(params: any) {
return (val: number, info: ValidationInfo) => {
const ambTemp = info.funcCall.inputs['ambTemp'];
const initTemp = info.funcCall.inputs['initTemp'];
return makeValidationResult({
errors: [
...(val < ambTemp) ? [makeAdvice(`Desired temperature cannot be less than ambient temperature (${ambTemp}). \n`, [
{actionName: 'Set desired equal to ambient', action: () => info.funcCall.inputs['desiredTemp'] = ambTemp }
])]: [],
...(val > initTemp) ? [`Desired temperature cannot be higher than initial temperature (${initTemp})`]: [],
]
});
};
}
//input: double desiredTemp = 30 {caption: Desired temperature; units: C; category: Environment; validatorFunc: Compute:DesiredTempValidator; }
This function accesses other inputs' values via the info
object.
It compares the validated input using contextual information.
If validation fails, it returns an error and possible action to make validation pass
(structure with actionName
and action
fields). You may make your validation interactive
and educational using such errors, warnings, and proposed actions.
We suggest you using functions from packages instead of scripts for easier code management.